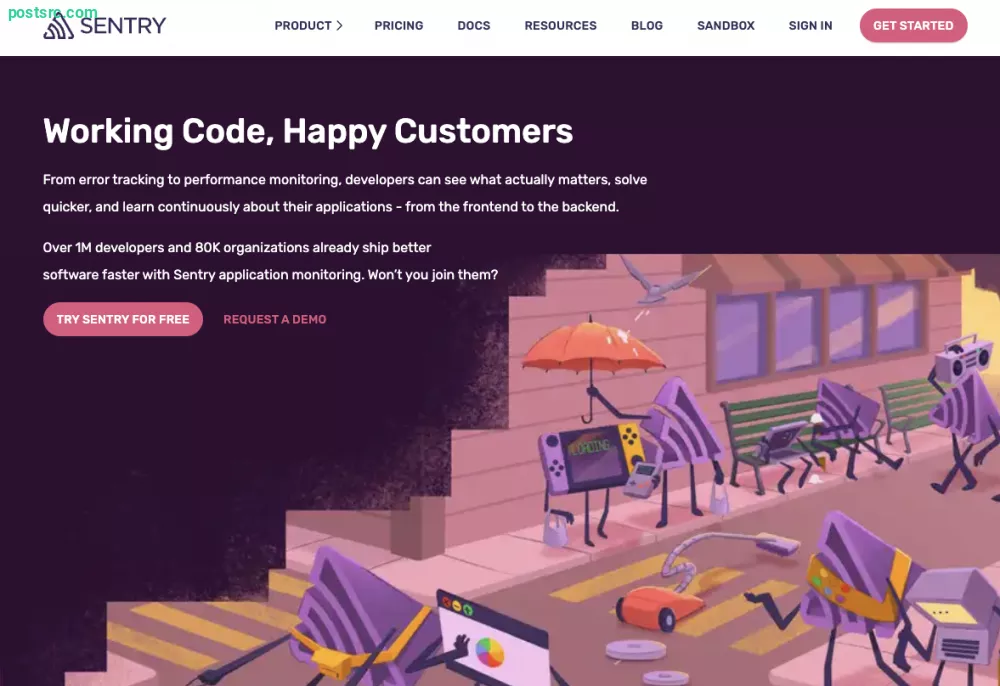
In this short snippet, you will learn how to set up Sentry which is "an Application Monitoring and Error Tracking Software" to your Laravel Nova. The steps are quite straight forward so let's get started.
Step 1: Install Sentry Package
First, you have to install the Laravel Sentry Package available on GitHub using Composer.
composer require sentry/sentry-laravel
Step 2: Setup Sentry
Once you have done that now you can set up the Sentry by publishing the configuration file using the command below. Do note that the "--dsn=" is unique to your project so you need to register for an account from the Sentry website.
php artisan sentry:publish --dsn=https://[email protected]/0
SENTRY_LARAVEL_DSN=https://[email protected]/0
Step 3: Verify Sentry Setup With Artisan
To verify the setup you can run the sentry:test command below and it will send a test event to the Sentry dashboard.
php artisan sentry:test --transaction
Step 4: Configure Nova For Sentry
Next, you need to configure Nova to send all its errors to Sentry. Nova uses its own internal exception handler instead of using the default App\Exceptions\ExceptionHandler. If you need to integrate third-party error reporting tools with your Nova installation, you should use the Nova::report. Typically, this method should be invoked from the register method of your application's App\Providers\NovaServiceProvider class:
App\Providers\NovaServiceProvider;
<?php namespace App\Providers; use Laravel\Nova\Nova; use Laravel\Nova\Cards\Help; use Illuminate\Support\Facades\Gate; use Laravel\Nova\NovaApplicationServiceProvider; class NovaServiceProvider extends NovaApplicationServiceProvider { /** * Register any application services. * * @return void */ public function register() { Nova::report(function ($exception) { if (app()->bound('sentry')) { app('sentry')->captureException($exception); } }); } }