<?php // request object $request->ip(); // helper method request()->ip();
Create Controller
This generally can be called from within a controller class and below is the full code example on how to achieve this.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class PostController extends Controller { public function index(Request $request) { dd($request->ip()); } }
<?php /routes/web.php Route::get('getclientip', [\App\Http\Controllers\PostController::class, 'index']);
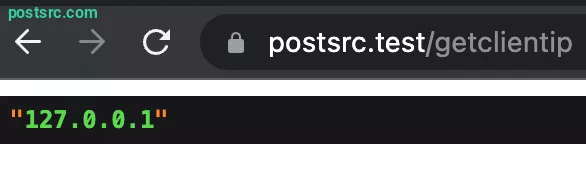
If you are still getting 127.0.0.1 as the IP, you need to add your "proxy", but be aware that you have to change it before going into production! For more details do read "Configuring Laravel Trusted Proxies". And add the following code to the TrustProxies class.
class TrustProxies extends Middleware { /** * The trusted proxies for this application. * * @var array */ protected $proxies = '*';
Leave a reply